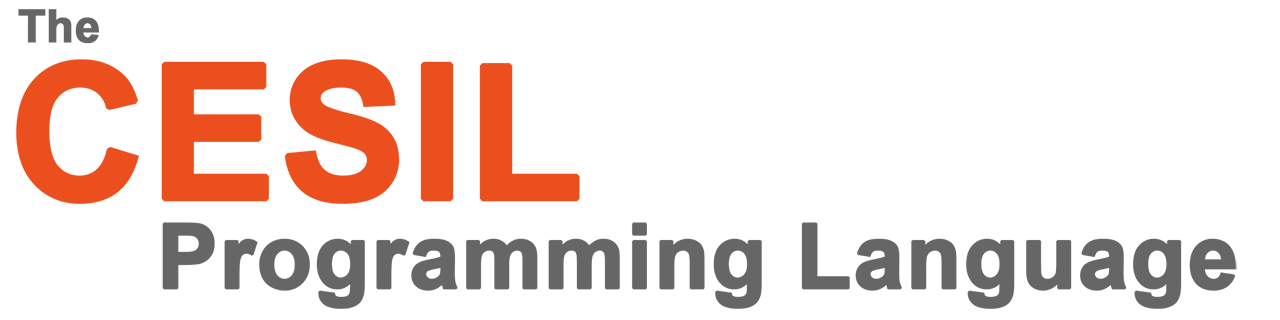
CESIL.org is an unofficial site dedicated to the CESIL programming language, and the home of my implementation of a CESIL interpreter, as well as my extended version of CESIL called CESIL "Plus".
What is "CESIL"?
CESIL is the Computer Education in Schools Instruction Language. It was created as an introductory instructional language, targeted at British secondary school students (ages 11 to 16). Developed by International Computers Limited (ICL), a sort of "British version of IBM", it was part of the "Computer Education in Schools" (CES) project, and was introduced in 1969.
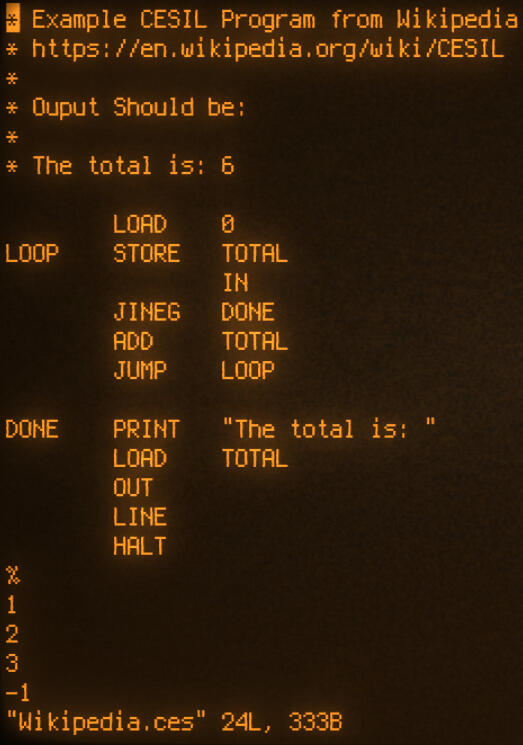
Example CESIL code from Wikipedia
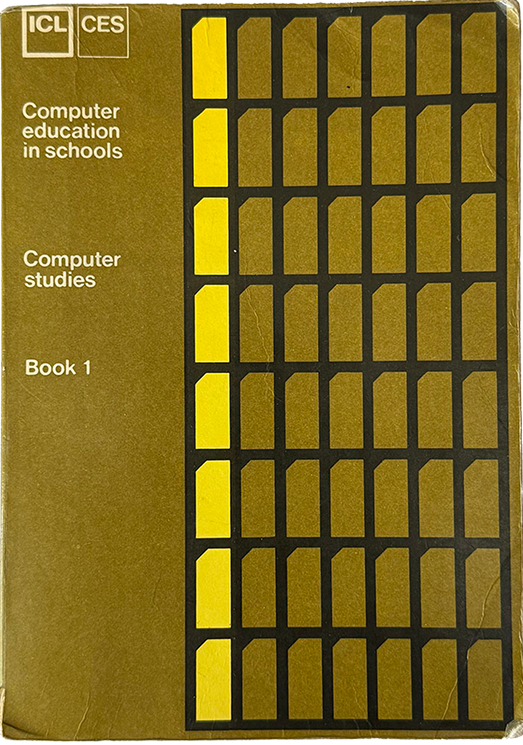
My original ICL CES Computer Studies - Book 1 (BASIC & CESIL)
The CESIL Language
Architecture
CESIL is a stack-less, non-interactive, assembly-like, language. It consists of 14 instructions which operate on 24-bit signed integers (-8388608 to +8388607), by way of a single accumulator.All values are purely scalar; there is no support for structures or arrays.The stack-less nature of CESIL means that, in addition to no user-addressable stack, there is no call-stack. Thus, CESIL has also no notion of functions nor of subroutines.The non-interactive model means that all data must be provided as part of the program's data section, as the time the code is executed.
Structure
CESIL programs consist of a sequential series of instructions, or lines of code, which are divided into three columns:The first column is an (optional) LABEL. The second column is the INSTRUCTION or operation. The third column contains the OPERAND (not all instructions require an operand):[LABEL] INSTRUCTION [OPERAND]OPERANDS, where present, can be a constant numeric value, a LABEL - which either represents a variable or a branch-target (position/line of code in the program), or a STRING (denoted by double quotes; only used by the PRINT instruction).LABELS, either for variables or for code lines, are alphanumeric, may be up to six characters long, and must begin with a letter.
Execution
Lines of code are executed sequentially, from the top of the program, until either the end of the program is reached or a flow-control instruction redirects it.Flow control consists of conditional and non-conditional "jump" operations; conditions are checked against the ACCUMULATOR and the target of the jump is provided by a LABEL.Data is read from the data section, one value at a time, when the IN instruction is executed. If no more data exists (all values have been read), an error occurs.Execution terminates when the program executes the HALT instruction, there are no more lines of code to process, or if an error occurs.
Instructions
CESIL comprises 14 instructions. In broad categories, these manipulate the value of the ACCUMULATOR (load, store, four-function math), control program flow or input/output data.In addition to "Computer Studies - Book 1", students were typically given (their own) language reference card. This covered both the BASIC and CESIL languages used in the CES program.The CESIL portions of this reference card are shown below, and serve as both the list of, and definition for, each of CESILs instructions here:
Coding
Coding, in CESIL, was not an interactive process. Code was either entered via Teletype or a local Console. Due to the nature of the machines that ran CESIL, which were ICL Mainframe Computers (typically 19XX and later 29XX series machines), they were not physically present in schools - usually reserved for a college, university or time-shared with a business.Students would write their code out, by hand, on pre-printed "Coding Sheets" (see below), and these would be sent to a central point (in my case, it was Nene College), to be entered by an operator - ready to be run with the rest of that school's programs.
A reproduction of my first CESIL program and coding sheet (the original is too scruffy to scan).
Running
Once programs had been submitted and entered, they would be run - again centrally. The results would be printed out, and then returned to us - usually in time for our next practical class (a week).If the code ran, you would see your results (if any). If not, your printout would consist of the errors that occurred, and any other output from the program.Since the turn-around time from submission to results was about a week, sometimes two, and the fact it was 100% non-interactive, made attempts at "debugging" the code quite interesting.One side effect of this was the practice of dry-running the code, multiple times, to find as many issues as possible before sending the code off to be executed. The coding sheets we used had columns to help facilitate this, as it was a mental/paper based exercise.
My CESIL and CESIL "Plus" Implementations
Where to Get Them
For those who just want to play with my implementations, these CESIL and CESIL "Plus" links will take you straight there. They point to the same repository, and the same code, as my CESIL "Plus" implementation is implemented in my main CESIL interpreter, and is simply enabled with a command-line option.
CESIL vs. CESIL "Plus"
"My" version of CESIL is an interpreter (the original was compiled/assembled), written in Python, which aims to follow the standard ICL implementation as closely I can with the documentation I have available (any errors in behavior are almost certainly mine).
It supports text files (whitespace delimits columns) and punched card files (fixed column positions) as input, runs from the command line, and includes a basic "debugger", so you can step through code one line at a time, and see the state of the accumulator, state flags and variables.
What is CESIL "Plus"?
CESIL "Plus" is my entirely unofficial take on an expanded version of the CESIL language. It adds twelve new instructions that expand the capabilities of the CESIL in ways that allow it to do things that are not possible in standard CESIL (e.g., interactive input, character output) and/or that make for easier, and more flexible coding (e.g., true subroutines, a stack and so on).
CESIL "Plus" Instructions
The additional twelve instructions for CESIL "Plus" are used, and behave, as defined below. Where an "operand" is specified, either literal constants or variables may be used:
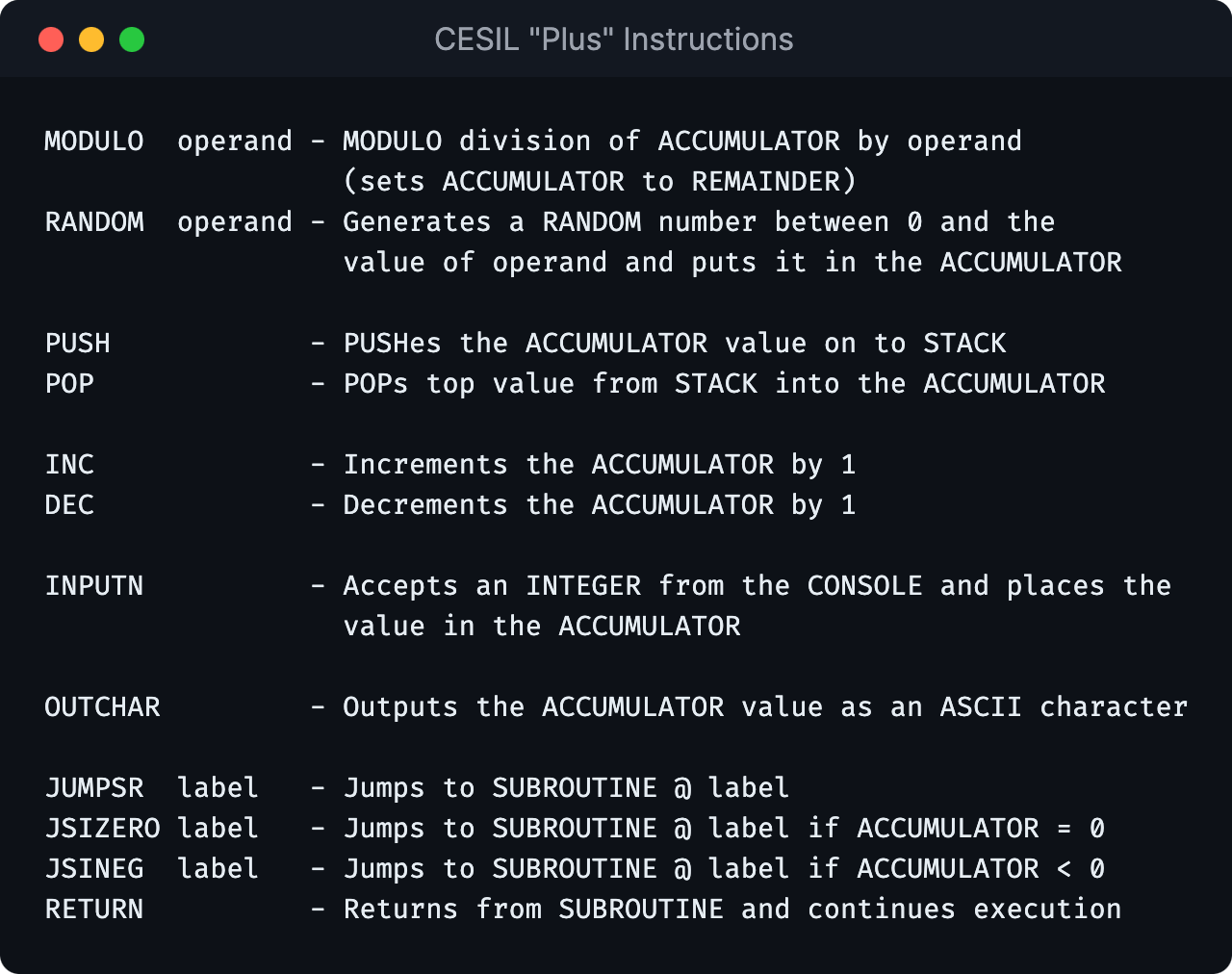
CESIL "Plus" Examples
To illustrate a couple of the features/additions that are part of CESIL "Plus", here are a couple of simple programs utilizing some of the new instructions.
sub_test.ces" - Uses some of the subroutine instructions (JUMPSR, RETURN) to demonstrate the use of subroutines works at a syntactic and behavioral level (nothing creative or clever).
"stack_test.ces" - Illustrates the use of the new STACK and its supporting instructions (PUSH, POP), again purely at a syntactic and behavioral level.
The combination of these capabilities make it much easier to address more complicated logic in CESIL, without resorting to the programmer having to do things like create large, hard to follow, conditional chains, to reuse code in a subroutine-like fashion (thus be able to return to the originating line of code).
Subroutine Example (CESIL "Plus") - sub_test.ces
Exanding the use of the CESIL "Plus" instructions, it is possible to create simple interactive programs and add non-numeric output (ASCII characters).
The screenshot, below, is from a very simple interactive "Golf" (putting) "game" I created to exercise the features of the "Plus" version of CESIL. It uses interactive input (INPUTN), random numbers (RANDOM), subroutines (JUMPSR, RETURN) and character output (OUTCHAR):
Why CESIL?
CESIL was a language I learned in school, in keeping with its CES origins, as part of the curriculum for my upper-school Computer Studies class ("O-levels" at the time, though CESIL was not part of the O-level syllabus).
As I was already coding in 6502 and Z80A assembly languages, as well as a BASIC and LOGO, it was a bit of a curiosity - but also a necessity for the classwork. The very limited nature of the language made me think about problems in a different way, which was academically interesting as well as oddly enjoyable.
I suppose nostalgia, with its propensity to make things seem much more fun/interesting than the reality of the time, is also partly at work here. I looked at some of the other open-source CESIL implementations, and they were fun to play with for a little bit. But it was really creating a CESIL interpreter (originally it was "compiled") that was fueling my interest.
Mentoring
As part of my on-going computer science/engineering/programming mentoring efforts, I wanted to introduce my mentees to a very simple, limited, programming model and get away from massively powerful functions riding on top of multiple levels of abstraction. Have them write some code that way, compare and contrast it with modern, higher-level, approaches.
And then, just as importantly, have them implement a similar language themselves.
From there, I wanted something to use in additional mentoring to look at ways of making code maintainable and both easily and cleanly extensible - which is what drove this "final" version (vs. earlier prototypes).
Extend, Compare & Contrast
Finally, to use the extension mechanisms from above, add additional features/instructions/capabilities (e.g., a stack, modulo division and subroutines) and see what affect those would have on the way code is written to solve different problems.
Resources, References & Links
A collection of other resources, reference material and links to other sites relating to the CESIL programming language, as well as the ICL Computer Education in Schools program, the machines CESIL originally ran on, example programs and other relevant information.
CESIL Implementations
Various other (i.e., "not mine", for which see above) implementations of CESIL exist today, including web-hosted versions and environments, tools, compilers and interpreters that can be run locally:
Interactive CESIL - Bovett Computer Services
This is a fully interactive CESIL programming environment including code editor, compiler, debugger and execution engine. It runs in your browser, is written in vanilla JavaScript, includes various sample programs, and is an excellent way to explore the CESIL language.Visual CESIL - Andrew John Jacobs (archived via Wayback Machine)
Another CESIL programming environment, also including an editor, compiler, debugger, and example programs. This is a C#/.NET 2.0 application for Windows.Wyrm CESIL - Wyrm Software (via the Google Play Store)
Wyrm CESIL is an interpreter for the CESIL language built for the Android platform.CESILPy - James Smith (via GitHub)
A CESIL interpreter written in Python. Runs from the command line, and includes a number of example CESIL programs.
Example CESIL Programs
Example programs written in CESIL covering simple illustrations through programs that push the limits of what CESIL could accomplish (at least within the confines of reasonable program lengths).
NOTE: Some links here go directly to source files (usually with a .ces extension), others to pages containing the program text (often documented and explained), others go to programs or pages that allow you to load examples from an application or download them as part of other software:
Hello_world.ces - One of myriad examples
The ubiquitous, mandatory, classic "Hello,. World!" ... in CESIL.Total Integers - Wikipedia Example - Wikipedia
Wikipedia's simple example program; totals a list of integers provided in a CESIL data section.Multiplication_Tables.ces - Ian Dunmore
Outputs multiplication tables based on the number of tables and number of multiplicands for each table as supplied in the CESIL data section.99Beers.ces - Marinus Oosters
Outputs the lyrics to "99 Bottles of Beer on the wall".Easter.ces - S. Robinson
Calculates and displays the date for Easter, for each year specified in the data section.
Example CESIL "Plus" Programs
The following examples depend on the CESIL "Plus" extensions (requires the -p or --plus options), in my CESIL interpreter implementation:
Golf.ces - Ian Dunmore
A simple interactive "Golf" (putting) game that illustrates, and exercises the extension instructions provided by CESIL "Plus".Sub_test.ces - Ian Dunmore
Demonstrates the syntax for, and use of, subroutines in CESIL "Plus".Stack_test.ces - Ian Dunmore
Illustrates the stack manipulation instructions and their application in CESIL "Plus".
CESIL Articles
Articles specifically addressing CESIL as a programming language, as well as its place in the Computer Education in Schools program:
CESIL - Wikipedia Page - Wikipedia
Wikipedia's overview of the CESIL language, it's history, illustration of it's instructions, an example program, and of course many links to other related topics, pages, sites and references.CESIL - An Introduction - D. Straker (in Liverpool Software Gazette, 2nd Ed., January 1980)
A brief introduction to CESIL, it's purpose, how the language is structured and works, coverage of its instructions via a fully narrated example program.
CESIL Artifacts
Downloadable/printable artifacts pertinent to CESIL, including scans of original documentation and reference cards as well as recreations of other materials:
CESIL - Coding Sheet - Recreation/Facsimilie
This is my recreation of the "Coding Sheet" we were given to write CESIL programs on. I've kept it as close to the original as I could, including the original copyright information.CESIL and BASIC Reference Card - Scans of the CESIL Pages
This is a PDF containing just the CESIL-specific pages of the reference card. You can find the full nine-page version (which includes BASIC), as individual scans, at ICL-CES.uk.
ICL Computer Education in Schools (CES)
A collection of sites and articles regarding the broader ICL Computer Educational in Schools (CES) program. This includes topics regarding CESIL as well as the general CES program, and some commentary on general computer education in British schools:
ICL-CES UK - David Bonham
The most complete coverage of the ICL CES program I have found, including details of the program itself, it's content, the systems employed, official publications, exam material and school work, details about CESIL and BASIC as used in the CES program, and a trove of other, well organized, ICL CES related material.ICL-CES 1969-1983 - Steve Rich
A broad array of ICL-CES material, including its history, interviews with some of the key figures in the ICL CES program, references to salient books, long-form articles and ICL CES newsletters, as well as links to other ICL and CES related topics.CES Enters Second Decade (Article) - Ian Sewell (in the ICL CES Newsletter #34)
An article on the current, and coming, state of, and developments in, Computer Education in Schools as per the ICL CES program.Shutdown or Restart? (Report): The way forward for computing in UK Schools - The Royal Society
A fascinating and extensive (>120 pages) report by Steve Furber (ICL Professor of Computer Engineering in the School of Computer Sciened at the University of Manchester, England) analyzing the current state of Computing education in schools and sets out a way forward for improving on the present situation.
Notice to Copyright Holders
Attempts to identify and/or contact current copyright holders for assets such as the "CESIL Reference Card" and the CESIL "Coding Sheet" (reproduced from scratch as a visual/content facsimilie of the original - including original copyright notice) were unsuccessful.I believe my usage/recreation of these assets falls under fair-use, for non-profit educational and/or documentary/editorial purposes.However, if said copyright holders have any issue with their reproduction here, I will be happy to remove these assets, modify their presentation, and/or transfer ownership (including the CESIL.org domain), at their behest.